A Complete Guide to Configuring PHP for Laravel
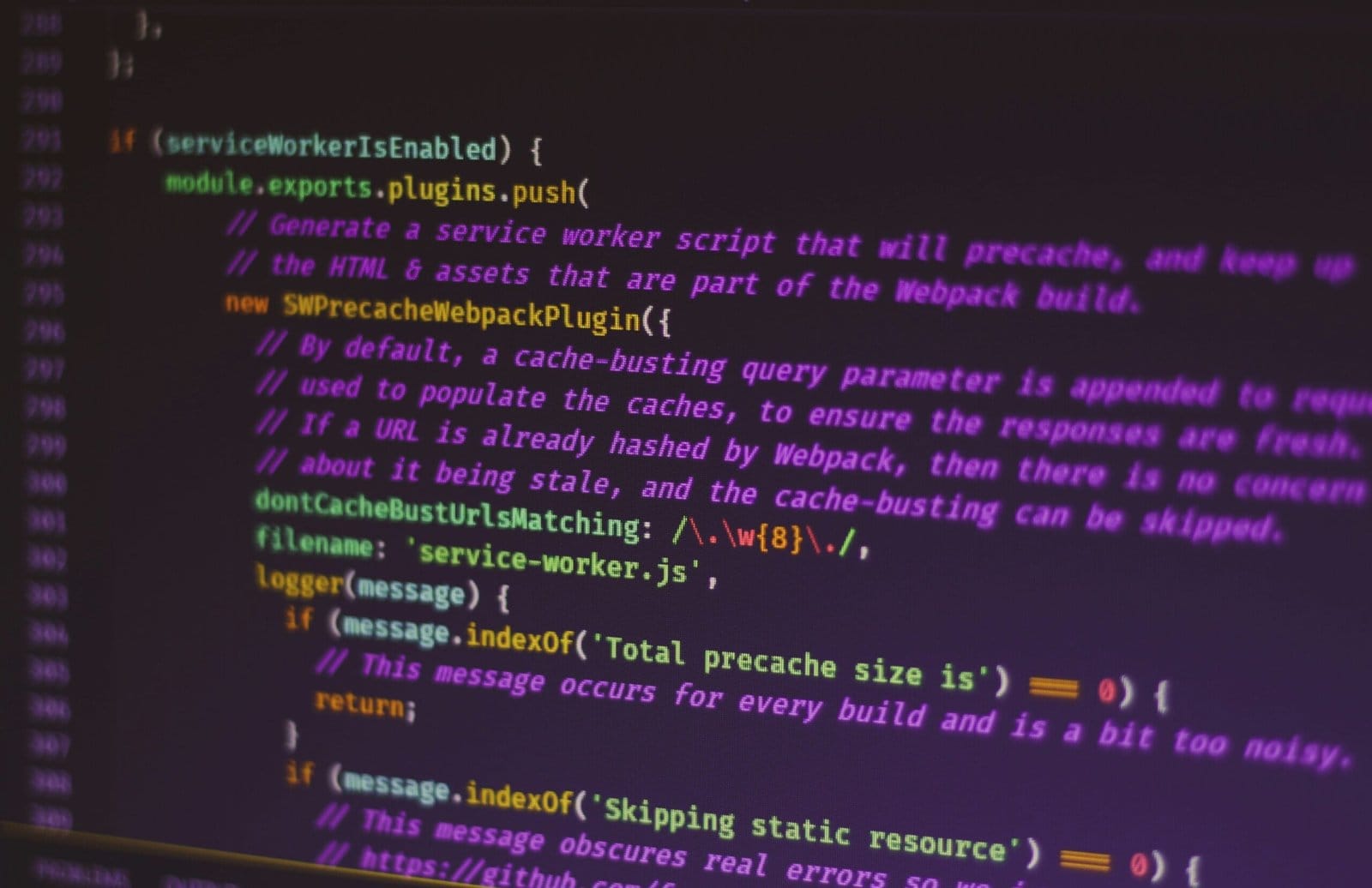
Guide to Configuring PHP for Laravel
Laravel is a highly regarded PHP framework designed to build robust applications with elegance and efficiency. It simplifies common tasks such as routing, authentication, and session management, enabling developers to focus on delivering high-quality code rather than dealing with repetitive tasks. One of the key aspects of working with Laravel lies in its reliance on optimal PHP configuration. Properly configuring PHP is essential for ensuring that Laravel applications run smoothly and efficiently.
When deploying a Laravel application, several prerequisites must be met to guarantee optimal performance. These include having a compatible version of PHP installed, as well as the necessary PHP extensions such as OpenSSL, PDO, Mbstring, Tokenizer, and XML. Each of these elements plays a vital role in ensuring that Laravel can leverage its full potential. Furthermore, adhering to best practices in PHP configuration can lead to improved application performance, security, and maintainability.
Effective PHP configuration goes beyond merely installing the required software. It involves tuning various PHP settings to align with the application requirements, optimizing memory usage, adjusting error reporting levels, and configuring the execution time limits. A well-configured environment ensures that Laravel can handle significant traffic while maintaining responsiveness and robustness. Individuals working with Laravel should therefore dedicate particular attention to the PHP configuration, as it ultimately influences the framework’s capabilities.
This guide will provide a comprehensive overview of the configuration process necessary for Laravel applications. Readers can expect to gain insights into the optimal PHP settings, implementation techniques, and best practices for maintaining performance. By the end of this guide, developers will be better equipped to configure PHP effectively, ensuring their Laravel applications meet both performance and reliability standards.
System Requirements for Laravel
To ensure optimal performance and successful deployment of Laravel applications, it is essential to meet specific system requirements. Laravel is a PHP framework that enables developers to create web applications efficiently. The first critical requirement is the version of PHP. As of October 2023, Laravel 9.x requires PHP 8.0 or later. Ensuring that you are running the appropriate version of PHP will allow you to take advantage of the latest features and improvements in the framework.
In addition to the PHP version, several PHP extensions must be enabled for Laravel to function effectively. These extensions include OpenSSL, PDO, Mbstring, Tokenizer, XML, Ctype, JSON, and BCMath. Each of these extensions provides essential functions that Laravel relies on, enhancing its capabilities and overall performance. Without these extensions, you may face significant issues when running your application, leading to potential failures and degraded performance.
Furthermore, Laravel relies on Composer, a dependency management tool for PHP. Composer simplifies the management of libraries and dependencies required by Laravel, ensuring that developers can seamlessly integrate third-party packages. Installing Composer is necessary, as it allows you to manage the libraries used in your Laravel application with ease.
As for the recommended web server options, Laravel can be run on servers like Apache and Nginx. Apache is widely used and has robust documentation available. Nginx, on the other hand, is known for its high-performance capabilities, making it a popular choice for modern web applications. It is advisable to use the latest versions of these web servers for enhanced security and performance.
In conclusion, adhering to the system requirements outlined above, including the correct PHP version, necessary extensions, Composer installation, and suitable web server selection, greatly improves the chances of running Laravel applications successfully while achieving optimal performance.
Installing PHP on Your Server
Installing PHP is a crucial first step in setting up a Laravel application. The process varies slightly depending on the operating system of your server. Below, we outline the steps for Windows, macOS, and Linux to ensure your PHP environment is correctly configured for Laravel.
For Windows, download the latest PHP version from the official PHP website. Choose the appropriate thread-safe version for your system and extract the files to a directory, preferably C:PHP. Next, you will need to add PHP to the system PATH. You can do this by navigating to System Properties > Advanced > Environment Variables, and then editing the PATH variable to include the PHP directory. After this, you can verify the installation by running the `php -v` command in the Command Prompt.
On macOS, the easiest way to install PHP is through Homebrew, a popular package manager. First, ensure Homebrew is installed, then run the command `brew install php`. This command will install the latest PHP version along with some common extensions. After installation, ensure PHP is set up properly by checking the installed version using `php -v`. You may need to enable extensions like `mbstring`, `openssl`, and `pdo` in your `php.ini` file, which is typically located in `/usr/local/etc/php/[version]/`.
For Linux users, the installation process largely depends on the distribution. For example, Ubuntu users can install PHP using the following commands: `sudo apt update` followed by `sudo apt install php libapache2-mod-php` to get PHP and the Apache module. For necessary extensions, run `sudo apt install php-xml php-mbstring php-zip`. After installation, remember to restart your web server with `sudo systemctl restart apache2` or the appropriate command for your setup.
Regardless of the operating system, ensure that you configure your PHP environment to meet Laravel’s requirements by enabling needed extensions. This will typically involve editing the `php.ini` file and uncommenting or adding extensions such as `curl`, `mbstring`, and `openssl`. Proper configuration of PHP is essential for ensuring your Laravel application runs smoothly.
Configuring PHP Settings for Laravel
Optimizing PHP settings is pivotal for enhancing the performance and reliability of Laravel applications. The first crucial step involves configuring the php.ini
file. This file serves as the primary configuration source for PHP, and adjustments made here can significantly influence your application’s behavior. The directives you set in php.ini
directly affect the runtime environment of Laravel.
One of the first adjustments concerns the memory limit. Laravel applications can be resource-intensive, particularly when handling large datasets or complex operations. It is recommended to increase the memory limit directive, changing it from the default value (often 128M) to 256M or more, depending on your application’s requirements. This change ensures that memory exhaustion does not occur, especially in production environments.
Error reporting is another critical aspect that demands attention. Modifying the error reporting levels in php.ini
enables developers to capture and log errors effectively. For production environments, it is advisable to only log errors by setting display_errors
to Off
while keeping log_errors
at On
. Conversely, during development, you can afford to set display_errors
to On
, allowing immediate visibility of issues.
Time zone settings also play a significant role in running Laravel applications smoothly. Laravel relies on accurate date and time handling. Therefore, ensure that date.timezone
is set to your server’s local time zone (for example, date.timezone = "America/New_York"
) to avoid unexpected behavior related to date and time functions.
Other directives such as upload_max_filesize
and post_max_size
should be configured according to the size of files you accept from users. Ensuring these settings align with your application requirements is vital for seamless file uploads and processing. Each of these configurations contributes to a more stable and efficient Laravel environment.
Installing Composer and Managing Packages
Composer is an essential tool for dependency management in PHP projects, particularly when working with Laravel. To begin using Composer, it must first be installed on your system. The installation process varies depending on your operating system. For Windows users, downloading the Composer-Setup.exe file from the official Composer website will launch an installer that guides you through the installation. For macOS and Linux users, Composer can be installed using the terminal by running the following command:
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
php -r "if (hash_file('sha384', 'composer-setup.php') === 'your_expected_hash') { echo 'Installer verified'; } else { echo 'Installer corrupt'; exit 1; }"
php composer-setup.php
php -r "unlink('composer-setup.php');"
mv composer.phar /usr/local/bin/composer
After installing Composer, it is important to manage PHP packages effectively within your Laravel project. To do this, you can utilize several key Composer commands. The composer require command adds packages to your project, updating the composer.json
file with the desired dependency.
For instance:
composer require vendor/package-name
Moreover, you can specify package versions to ensure compatibility. For example, using:
composer require vendor/package-name:^1.0
will fetch any compatible version greater than or equal to 1.0. Additionally, autoloading is crucial in Laravel projects, allowing you to easily manage class dependencies without the need for manually including files. By including the autoload
section in the composer.json
file, you can define how classes within your project should be auto-loaded.
Maintaining your dependencies is equally important, and regular usage of commands like composer update can help keep packages current while ensuring that related dependencies are also updated harmoniously. Overall, mastering Composer is pivotal for efficiently managing packages in Laravel development.
Setting Up a Development Environment
Configuring a local development environment is crucial for efficiently running Laravel applications. Among the leading options for establishing a robust PHP development environment are Homestead, Valet, and Docker. Each of these solutions offers unique advantages, allowing developers to choose one that best fits their workflow.
Laravel Homestead is a Vagrant box designed specifically for Laravel. It provides a pre-packaged environment, making it easy to set up a development system that comes preconfigured with PHP, Nginx, MySQL, and other necessary tools. To get started with Homestead, first, ensure that Vagrant and VirtualBox are installed on your machine. After that, follow these steps: download the Homestead box, configure the `Homestead.yaml` file to match your project needs, and run `vagrant up` in the terminal. This process automatically sets up a virtual machine that can run your Laravel applications seamlessly.
Another popular option is Laravel Valet, particularly for developers using macOS. Valet offers a minimalist setup for a local development environment, allowing developers to serve sites instantly without needing to spend time configuring complex software stacks. To install Valet, ensure that you have Homebrew and Composer installed, then run the command `composer global require laravel/valet`. Once Valet is installed, link your project directory using `valet park`, and your Laravel application will be accessible via a local domain.
For those who prefer containerization, Docker is an excellent option. Docker allows developers to create uniform environments that are both portable and easy to manage. Begin by creating a `docker-compose.yml` file in your Laravel project root, specifying the necessary services such as PHP, Nginx, and MySQL. After setting up the file, run `docker-compose up` to start your containers. This approach not only isolates dependencies but also ensures that you have the same environment across all development machines.
Choosing the right development environment is essential for smooth Laravel application development. Homestead, Valet, and Docker each provide distinct features tailored to different requirements, allowing for effective PHP configuration and development workflow.
Using PHP Frameworks and Libraries with Laravel
Integrating additional PHP frameworks and libraries with Laravel can significantly enhance the functionality and performance of your applications. Laravel, known for its robustness and elegant syntax, provides a solid foundation for seamlessly integrating these external resources. However, understanding how to use these tools effectively is crucial for maximizing their benefits.
One popular library that works exceptionally well with Laravel is Guzzle. This PHP HTTP client simplifies sending requests to other web services and handling responses. To configure Guzzle within a Laravel application, you can leverage the built-in HTTP client that Laravel provides. This allows you to make API calls effortlessly, which is particularly beneficial for applications needing external data connections. To get started, simply include Guzzle in your project via Composer and utilize its methods to streamline your API interactions.
Another noteworthy library is Laravel Debugbar, which aids in debugging and profiling your Laravel applications. It is particularly useful for developers looking to optimize performance or troubleshoot issues. Installation is straightforward, requiring a Composer installation, followed by publishing the configuration file to tailor the output to your needs. Adhering to best practices, you should only enable debugging in your local development environment to avoid exposing sensitive performance data in production.
A comprehensive approach to dependency management is essential when integrating libraries. Using Composer ensures that all necessary dependencies are handled correctly and updates can be managed consistently. Additionally, you should consider using the service container of Laravel for binding libraries into the application, which promotes a cleaner separation of concerns and enhances maintainability. This approach enables you to resolve dependencies easily, promoting a modular structure that is well-suited for complex applications.
Thus, by thoughtfully selecting and properly configuring PHP frameworks and libraries, you can significantly extend the capabilities of your Laravel applications while ensuring an optimal development experience.
Debugging and Troubleshooting PHP Configuration Issues in Laravel
Developing applications using the Laravel framework often requires careful configuration of the PHP environment. However, developers may encounter various PHP configuration issues that can affect application performance and cause unexpected behavior. Understanding how to diagnose and troubleshoot these problems is crucial for ensuring a smooth development process.
Commonly, one of the initial hurdles developers face is related to outdated or conflicting PHP extensions. Laravel relies on certain extensions to function properly. For instance, the absence of the OpenSSL extension can lead to errors related to secure URL generation. To address this, developers should check their PHP configuration file (php.ini) and ensure that the necessary extensions are enabled. Running the command php -m
can also help identify loaded modules and spot any omissions in needed extensions.
Another frequent issue is related to memory limits defined in the PHP configuration. Laravel applications, especially those handling large datasets or heavy processing tasks, may hit memory limits, triggering fatal errors. Modifying the memory_limit
directive in php.ini or utilizing the ini_set
function in the application code can resolve this problem. It is recommended to test the application after each change to assess improvements in performance.
Debugging with Composer can also illuminate PHP configuration issues. Running composer diagnose
checks for common problems in the Composer setup, including PHP version mismatches or missing extensions that might hinder Laravel’s operation. Error logs, both from Laravel and PHP, are invaluable for troubleshooting. These logs provide insights into specific issues, serving as a guide for quickly diagnosing configuration problems.
Finally, implementing proper error handling in Laravel can help identify misconfigurations. Setting the APP_DEBUG
environment variable to true offers detailed error messages that can elucidate the underlying causes of PHP configuration problems. This allows developers to address and rectify issues more effectively, ensuring the Laravel application runs smoothly in its environment.
Best Practices for PHP Configuration in Laravel
Configuring PHP for Laravel applications requires careful consideration of several factors, including security, performance, and resource management. Adhering to best practices can enhance the overall efficiency and robustness of your application. First and foremost, security should be a priority. Ensure that you maintain the highest security standards by regularly updating your PHP version and applying relevant patches to minimize vulnerabilities. Additionally, configure your web server to prevent exposure of sensitive files, such as the Laravel configuration files, by restricting access.
Performance tuning is another essential aspect of PHP configuration in a Laravel environment. Utilize opcode caching mechanisms like OPcache to improve the performance of your application by storing the compiled PHP scripts in memory, reducing the need for repeated parsing. Moreover, enable HTTP/2 support if your server currently allows it, as it can significantly increase loading speeds and improve resource management.
When managing resources, it is crucial to optimize database configurations. Use Laravel’s Eloquent ORM effectively by implementing pagination and eager loading strategies, which help reduce memory usage and improve query performance. Additionally, consider employing queues for handling time-consuming tasks, such as sending emails or processing uploads, to keep your application responsive. Regularly monitoring your application’s performance through tools like Laravel Telescope or other profiling tools can help identify bottlenecks and optimize for resource usage.
Finally, ongoing maintenance strategies should not be overlooked. Establish a routine for checking error logs and performance metrics to identify any issues before they escalate. Regularly back up your application and its database to safeguard against data loss. By following these best practices for PHP configuration in Laravel, you contribute to ensuring that your application remains robust and efficient in the long run.